C3.2 Read and alter existing code, including code that involves sequential events, and describe how changes to the code affect the outcomes.
Skill: Reading Code
Reading code involves interpreting blocks or commands to deduce the meaning of code. This activity helps students make predictions about the behaviour of the code. Reading code is also an important part of debugging, which is the identification and correction of errors in code. By reading code, it is possible for students to find the reason(s) why the code is not working. This skill does not correspond to the computer reading and executing the code, but rather to the student reading code to verify it.
For example, students can read the following code and identify the pattern:
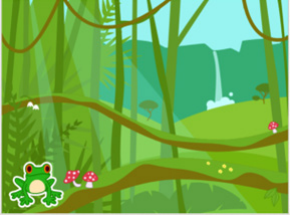
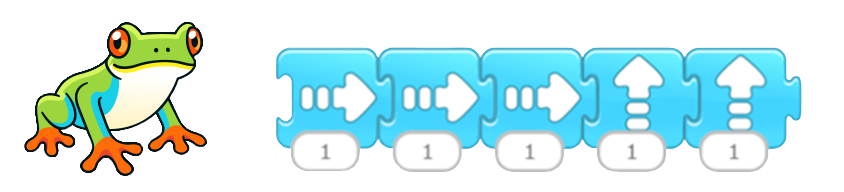
In the example shown above, students could predict the behaviour of the frog before executing the code. In this situation, executing the code would not work because a starting condition (the green flag) is missing. Therefore, the student might identify and correct this error prior to executing it.
Skill: Altering Code
One of the reasons to alter code is to save time by not starting from scratch but to use existing code to build something new. Altering code can also be done to make the code more efficient and reduce runtime or to demonstrate that there is more than one way to use code to represent a situation. Another reason to alter code is to fix errors and make it functional, a process called debugging.
For example, a code that places Canadian coins in a pattern might place nickels as follows:
5
5 5
5 5 5
5 5 5 5
5 5 5 5 5
5 5 5 5 5 5
Are there other possible codes to represent this same value?
The student could modify the code to extend the pattern or to replace the nickels with sprites of different coins that would represent the same values.
Example
5 10 5
5 10 10 10 25 25
Skill: Describing the Impact of Altering Code
Understanding the sequencing of events that will occur based on the code is important for altering code so that one can make predictions about how the changes will affect the outcome.
For example, sequential moves to the left followed by the same number of sequential moves to the right will result in a null move - the initial and final position of the sprite will not have changed. The student who understands this concept will be able to use moves in all directions to create series of 10 moves with a specific end point.
Example
A sprite must move two units to the right to be on top of another sprite, but the instruction is to create combinations of 10 moves. The student could make a move of six units to the right and four units to the left for a total move of 10 units, but the final position of the sprite is only two units away from its initial position.
This kind of exercise can be useful to link the learning of relative position with addition facts.
Knowledge: Sequential Events
The basis of all code is pattern. Sequential events are a set of instructions executed one after another, usually from top to bottom or left to right on a screen.
For example, in the map of a fictional city like the one shown below, one could start with a specific code and ask the student to predict the final location of the sprite or even modify the code so that the sprite could go to another location.
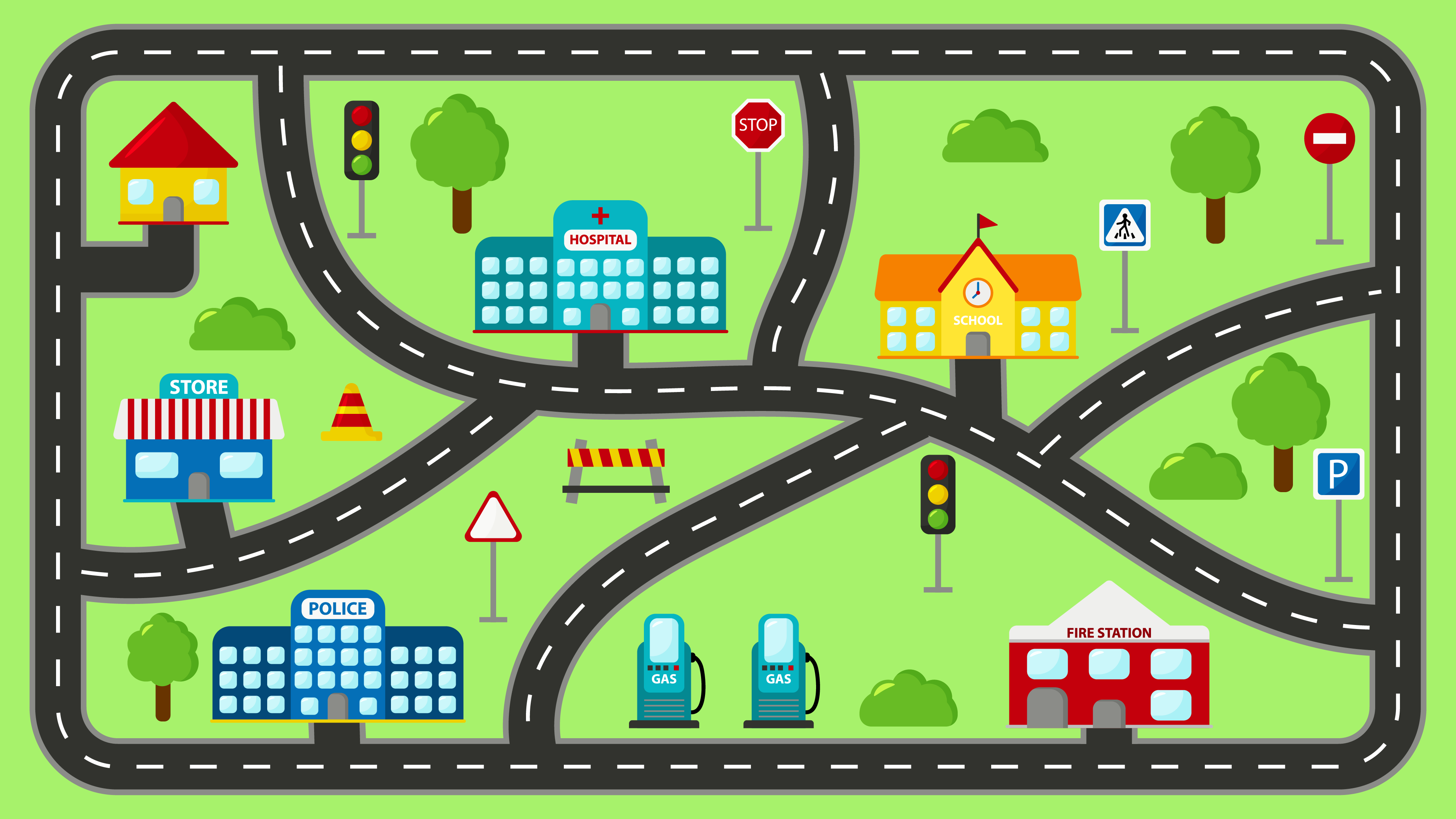

The pseudocode associated with this code might look like this:
Initial position = to the left of the yellow house
Starting condition = true
Move 5 steps
Direction = bottom ↓
Move 2 steps
Direction = right →
Final position = (unknown)
The student could predict, for example, that the final position of the sprite will be either at the store, the police station, or the gas station, since the sprite starts at the yellow house and moves down and to the right. They could then verify their prediction by running this code.