C3.1 Solve problems and create computational representations of mathematical situations by writing and executing code, including code that involves sequential, concurrent, repeating, and nested events.
Skill: Computational Problem Solving
Coding can be used to automate tasks and to represent and solve problems. By its very nature, coding lends itself well to trial-and-error learning, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "What will happen if…?".
The student, for example, may ask the question, "What will happen if I want to represent a repeating and a growing pattern?" To solve this problem, the student will need to use one loop to represent the repeating element of the pattern and a second loop to represent the growing element.
Example
Code (Scratch) |
Result |
![]() Source: Scratch Jr. |
![]() |
In this example, there is a loop nested within another loop. The outer loop shows the increasing element (two repeats at the second term in the pattern), while the inner loop represents the repeating element, that is, the five diamonds one over the other.
Here is the code enlarged to better see the loops:
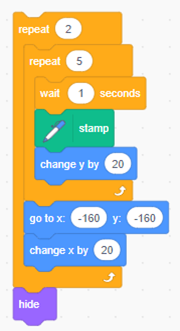
Skill: Representing Mathematical Situations Computationally
Coding can be used as a mathematical learning tool to represent and manipulate complex mathematical situations.
The student, for example, represent geometric shapes digitally by applying their geometric properties in their code. Loops can be used to repeat elements of a geometric shape as well as the repetition of shapes to create a design. Here is an example of code to create a rectangle and then to create a rectangle at each rotation:
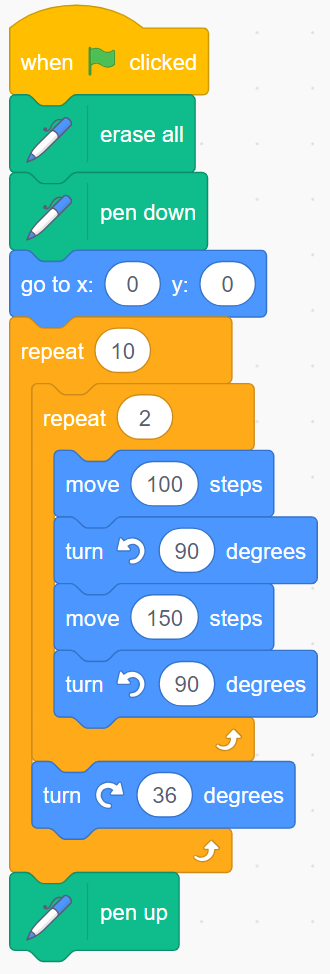
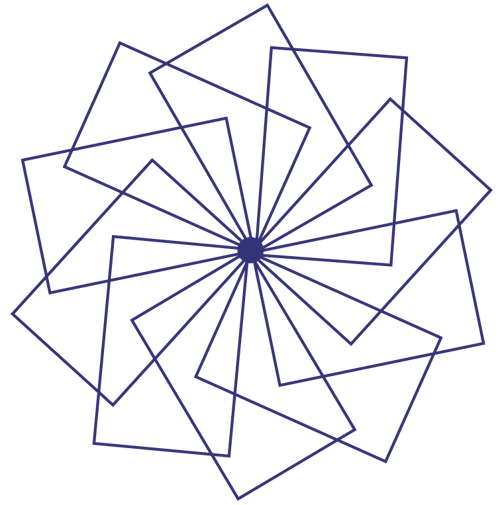
Skill: Writing Code
Writing and altering a code involves sequencing instructions in a specific order, following the syntax of a programming language. Writing code can be similar to writing text. Pseudocode is writing the instructions for a code in no particular program language.
The pseudocode below, outlines the process to calculate the area and perimeter of a rectangle.
Sprite Variables [base] = base of the rectangle [height] = height of the rectangle [area] = area of the rectangle [perimeter] = perimeter of the rectangle |
Starting condition = true |
Ask - "What is the base of the rectangle?" Value of [base] = response |
Ask - "What is the height of the rectangle?" Value of [height] = response |
Value of [area] = [base] * [height] |
Value of [perimeter] = [base]+[height]+[base]+[height] |
Say - "The area of the rectangle is [area]. " |
Say - "The perimeter of the rectangle is [perimeter]. " |
End |
This pseudocode can act as the planning or prewriting step. The resulting code might look like this:
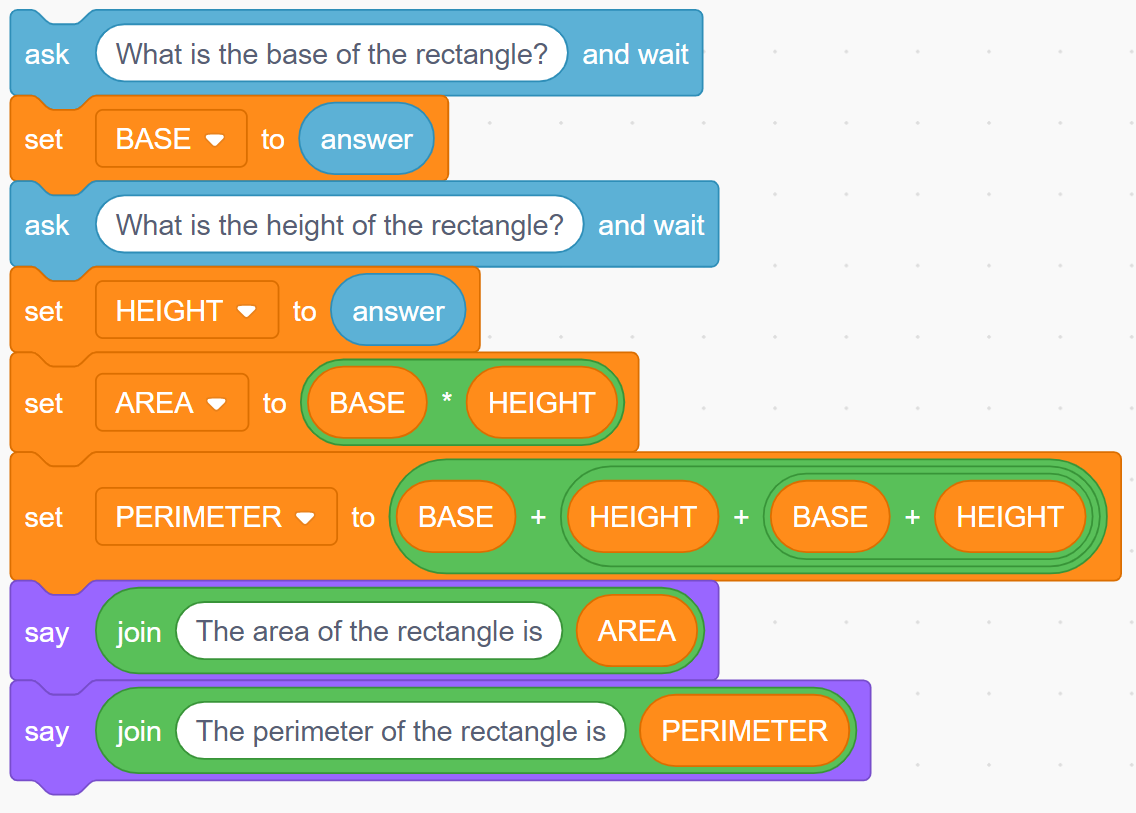
Skill: Executing Code
Execution of code is when the code is read and compiled by the coding program and is referred to as the output. In block coding, the execution of a code is often done by means of a button in the interface, while text-based programming languages require precise compilation software that essentially translates the code from the programming language to the machine language (for example, binary code) so that it can execute the code. This also applies to robotic devices.
Some examples of run buttons for block coding software:

Source: Makecode.
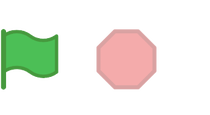
Source: Scratch Jr.
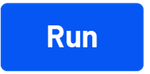
Source: Programiz
Knowledge: Nested Events
Nested events are control structures placed inside other control structures (for example, loops placed inside loops).
A "control structure" is any line or block of code that influences the way the code is executed. Control structures affect the flow of the program. They include repeating lines of code or selecting whether to execute specific lines of code. Sequence and repetition (loops) are control structures.
To understand the impact of changing the number of repetitions in each loop of a code with nested loops, the student could experiment with a variety of number combinations and record the results. Then the student could write a code and share it with the class, who would then predict the outcome of the code. For example, the student could use the code below and see the effect of a variety of numbers on the arrangement of apples.
The representation of sprites in an array becomes even more obvious when using nested loops to define the rows and columns of the layout.
Here is an example of code, for an array, that has nested events:
Example of code (Scratch Jr) |
|
![]() |
|
Result of the execution |
|
![]() |
The code now represents a multiplication using the rectangular layout by using a loop nested within another loop. The outer loop represents the number of rows, while the inner loop represents the number of apples per row. The multiplication can therefore be written as follows:
(number of outer loop repeats) × (number of inner loop repeats) or, in this example, 3 × 6.
Relevant Questions:
- What is the maximum number of apples that can be displayed on the screen?
- Is it possible to represent a prime number in a rectangular arrangement?
- How many different ways can the number 36 be represented?
- What would happen if a third loop was embedded in the code? Where would you place it?
Knowledge: Events and Structures (Prior Knowledge)
Sequential Events
A set of instructions executed one after the other.
Example
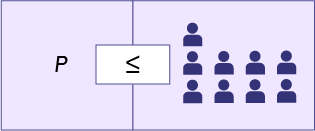
Simultaneous Events
Several events that occur at the same time.
Example
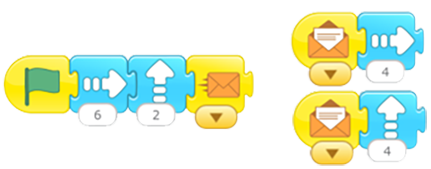
Recurring Events
Repeating events. In coding activities, loops are used in code to repeat instructions.
Example
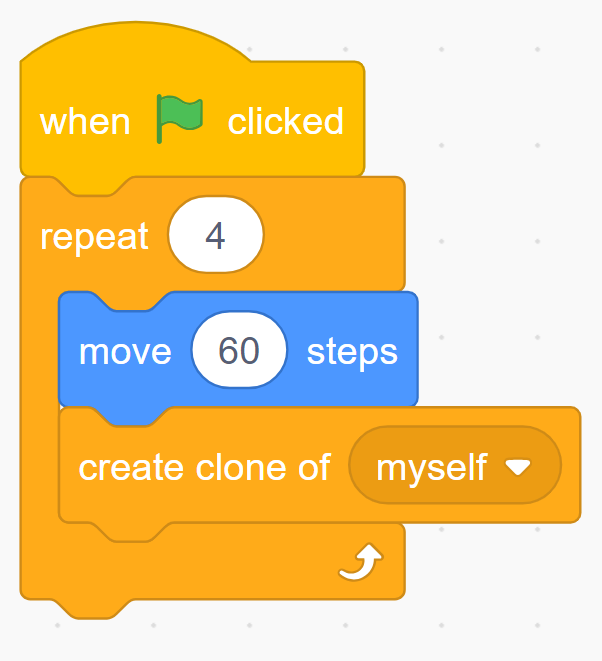