C3.1 Solve problems and create computational representations of mathematical situations by writing and executing code, including code that involves conditional statements and other control structures.
Skill: Solving Problems Using Computational Methods
Coding can be used to automate tasks and visualize mathematics to facilitate problem solving. By its very nature, coding lends itself well to trial and error, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "what will happen if…?".
In addition, the question "what will happen if…?" allows one to start a conversation about conditions and conditional statements. For example, in a probability problem, one might ask "what will happen if I roll a die 1,000 times?" or even "how many times will I get 2 by rolling a die 1,000 times?" Given the time required to perform this experiment manually, code becomes an efficient way to solve such a problem.
Skill: Representing Mathematical Situations in Computational Ways
Coding can be used as a representational tool in the same way as manipulatives. By using written blocks or commands, very complex mathematical situations can be modeled and manipulated visually, which can make very abstract concepts concrete.
For example, the representation of the die problem can be done with a code sequence that looks like this:
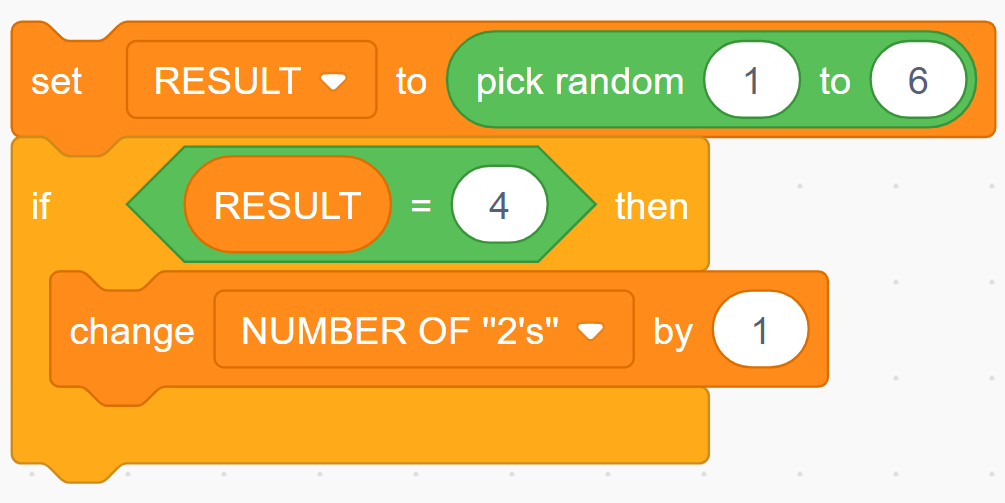
In this code, a random number from 1 to 6 is used to represent the action of rolling a die, and the conditional statement "if result = 2, then add 1 to the variable (number of "2")" essentially counts the number of times the number 2 is rolled.
The student could also associate a conditional instruction with a sprite to visually represent the faces of the die depending on the outcome.
Skill: Writing Code
Writing or editing a code consists of placing instructions in a specific order, following the syntax of a programming language. Writing code can be similar to writing a text. Pseudocode writing, on the other hand, consists of writing the code instructions in the familiar language. Block coding can make it easier to follow the syntax by using different block shapes and colors.
With the addition of conditional statements, for example, the student has several commands at his disposal, which makes it possible to make more complex codes. However, this requires the use of a certain syntax when writing the pseudocode. The student will first need to use spaces and indents strategically to represent the intent of the pseudocode and to facilitate transfer to code.
Here is an example of pseudocode in which suits are associated with the result obtained after rolling a die. Here, we use the "if, then, else" command to allow several possibilities.
|
SET "RESULT" TO (RANDOM NUMBER 1-6) |
|
|
|
|
|
By placing the statements(if, then, else) on a new line and indented, it will be easier to transform this pseudocode into code on the computer. Note that the pseudocode is intended to make it easier for the student to understand the code, so the exact structure may differ from student to student. The important thing is that the student can understand what is going on in the pseudocode.
Skill: Execute Code
Code execution is the step where the code sequence is read and compiled by the computer. It is at this stage that a functional code will give the desired result, or output (and a non-functional code will give a different output or no output). In block coding, the execution of a code is often done by means of a button in the interface, while text-based programming languages require precise compilation software that essentially translates the code from the programming language to the machine language (e.g., binary code) so that it can execute the code. This also applies to robotic devices.
Here are some examples of run buttons for block coding software:

Source : makecode
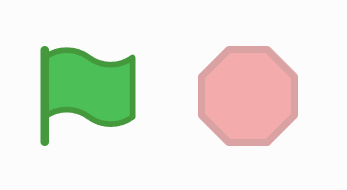
Source : Scratch Jr
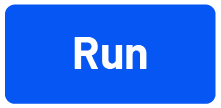
Source: Programiz
Knowledge: Conditional Instructions
A conditional statement is a type of coding instruction that instructs the computer to compare values and expressions and make decisions. A conditional statement tells a program to perform an action depending on whether the condition is true or false.
Conditional statements are first present when the execution of an event or a sequence of code depends on the achievement of a specific condition. Boolean values (true or false) are often used and associated with terms such as if, else, until and when.
The code allows the representation of multiplications using the rectangular layout and could be transformed to determine whether the layout represents a square or not. It would then be the following pseudocode:
If "value_1" = "value_2
So
Say "Yes, it's a square!"
Otherwise
Saying "No, it's not a square!"
Since we are talking about a geometric shape, the names given to "value_1" and "value_2" could be more precise, like "base" and "height". Here is a representative code example of this pseudocode:
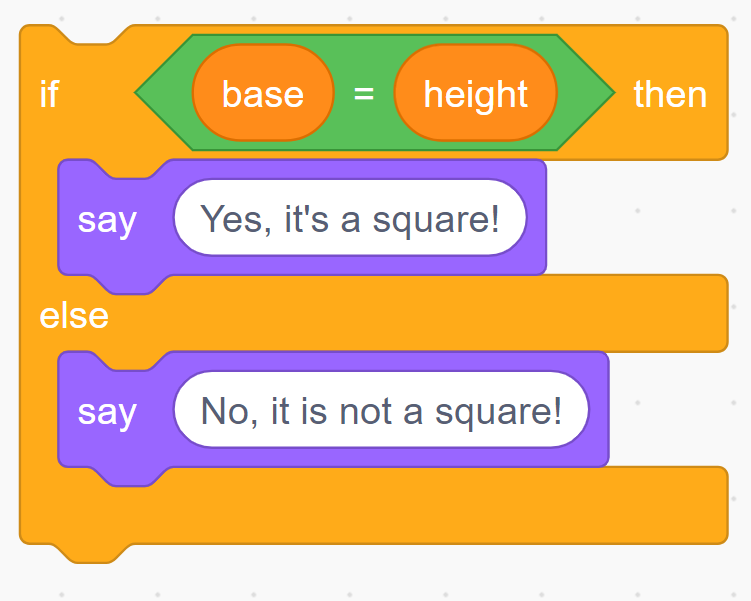
Knowledge: events and structures (prior knowledge)
Sequential events
A set of instructions executed one after the other, usually from top to bottom or from left to right on a screen.
Example
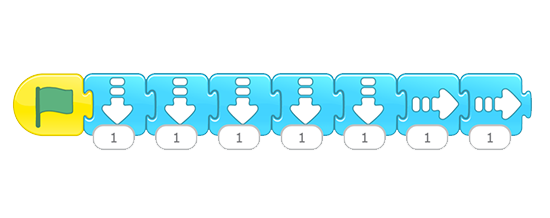
Simultaneous events
Several events that occur at the same time.
Example
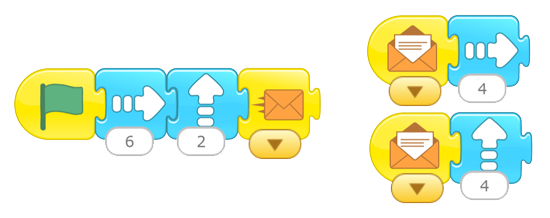
Recurring events
Repeating events. In coding activities, loops are used in code to repeat instructions.
Example
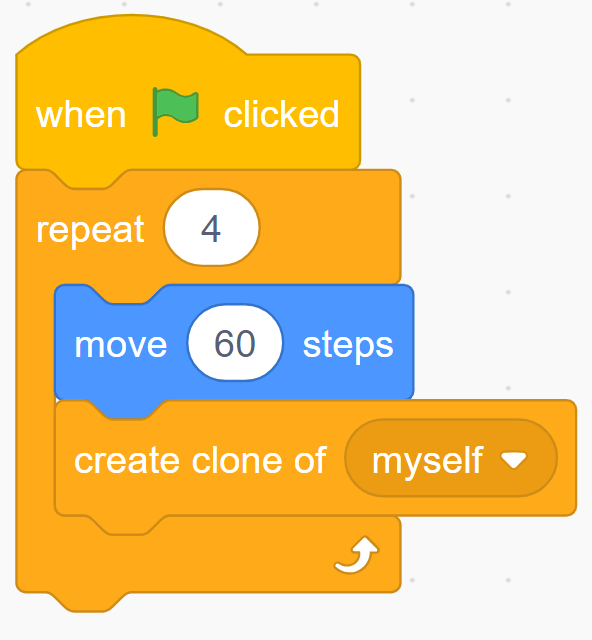
Nested events
Control structures placed inside other control structures. For example, loops occurring inside loops or a conditional statement being evaluated inside a loop.
Example
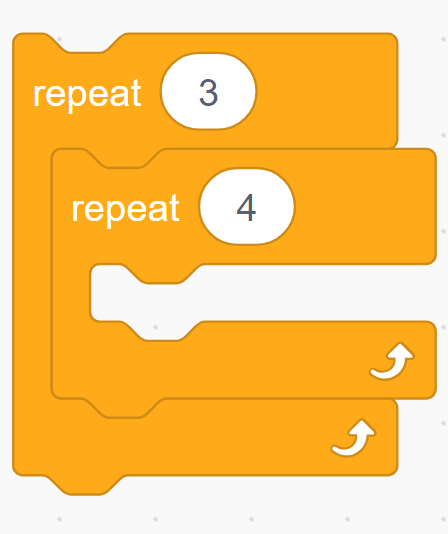