C3.1 Solve problems and create computational representations of mathematical situations by writing and executing efficient code, including code that involves conditional statements and other control structures.
Skill: Computational Problem Solving
Coding can be used to automate tasks and to represent and solve problems. By its very nature, coding lends itself well to trial-and-error learning, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "What will happen if…?".
In addition, the question "what will happen if…?" allows one to start a conversation about conditions and conditional statements. For example, in a probability problem, one might ask "what will happen if I roll a die 1,000 times?" or even "how many times will I get 2 by rolling a die 1,000 times?" Given the time required to perform this experiment manually, code becomes an efficient way to solve such a problem.
Skill: Representing Mathematical Situations Computationally
Coding can be used as a mathematical learning tool to represent and manipulate complex mathematical situations.
For example, it is possible to represent a mathematical situation in many ways using code, but some representations are more effective than others. A block-based coding program might, for example, help the student generate a list of data to identify its range, but a spreadsheet can accomplish the same task with less code. The student might first progress from a less efficient, but more visually understandable, representation to one that is more efficient, but has more abstract components and structures.
Code | Result |
---|---|
Block-Based Coding
![]() |
![]() |
Spreadsheet
![]() |
![]() |
Skill: Writing Code
Writing and altering a code involves sequencing instructions in a specific order, following the syntax of a programming language. Writing code can be similar to writing text. Pseudocode is writing the instructions for a code in no particular program language.
With the addition of conditional statements, for example, the student has several commands at his disposal, which makes it possible to make more complex codes. However, this requires the use of a certain syntax when writing the pseudocode. The student will first need to use spaces and indents strategically to represent the intent of the pseudocode and to facilitate transfer to code.
Here is an example of pseudocode to associate costumes to the result obtained after rolling a die. Here, we use the "if, then, else" command to obtain several possibilities.
Sprite - DIE Costumes: Variables: RESULT |
SET "RESULT" TO (RANDOM NUMBER 1-6) |
IF "RESULT" = 1 THEN PUT ON COSTUME: OR |
IF "RESULT" = 2 THEN PUT ON COSTUME: OR |
IF "RESULT" = 3 THEN PUT ON COSTUME: OR |
IF "RESULT" = 4 THEN PUT ON COSTUME: OR |
IF "RESULT" = 5 THEN PUT ON COSTUME: OR PUT ON COSTUME: |
The resulting code might look like pseudocode, but a good understanding of the use of variables reduces this code to only a few lines.
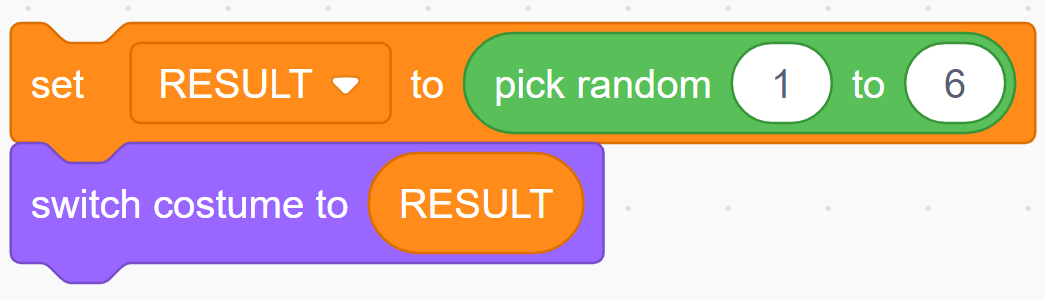
By placing the statements (if, then, else) on a new line and indented, it will be easier to transform this pseudocode into code on the computer. Note that the pseudocode is intended to make it easier for the student to understand the code, so the exact structure may differ from student to student. The important thing is that the student can understand what is going on in the pseudocode.
Skill: Executing Code
Execution of code is when the code is read and compiled by the coding program and is referred to as the output. In block coding, the execution of a code is often done by means of a button in the interface, while text-based programming languages require precise compilation program that essentially translates the code from the programming language to the machine language (for example, binary code) so that it can execute the code. This also applies to robotic devices.
Some examples of run buttons for block-based coding program
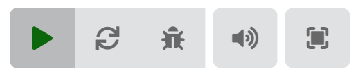
Source: makecode.
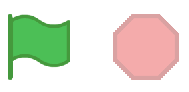
Source: Scratch Jr.

Source: Programiz.
Knowledge: Events and Structures (Prior Knowledge)
Sequential Events
A set of instructions executed one after the other.
Example
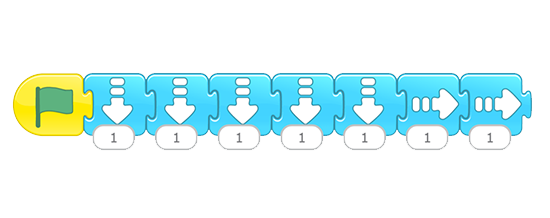
Simultaneous Events
Several events that occur at the same time.
Example
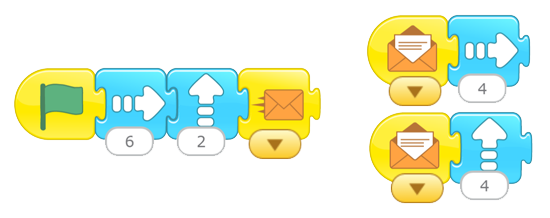
Recurring Events
Repeating events. In coding activities, loops are used in code to repeat instructions.
Example
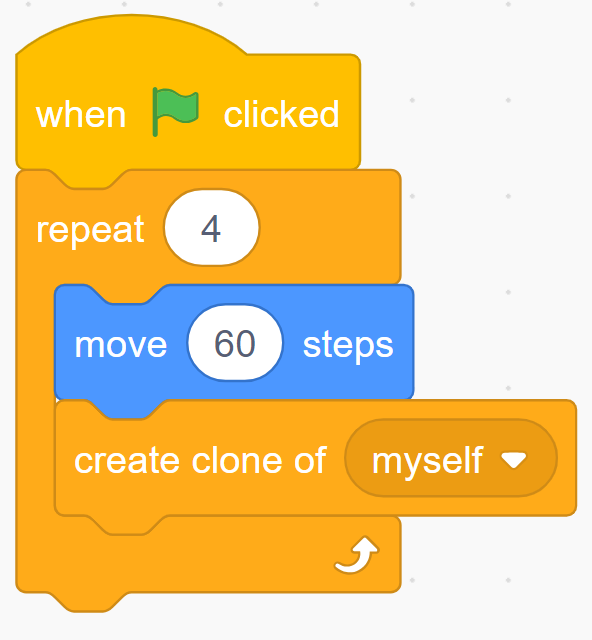
Nested Events
Control structures placed inside other control structures. For example, loops occurring inside loops or a conditional statement being evaluated inside a loop.
Example
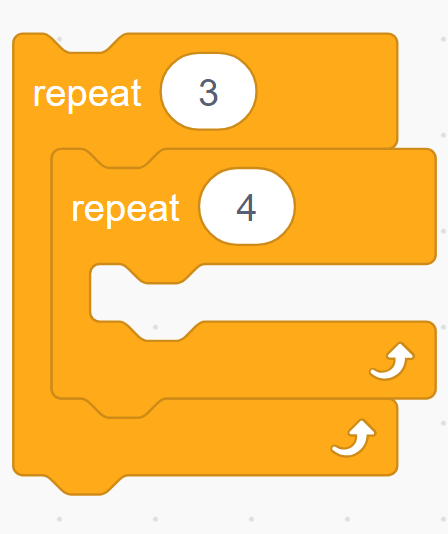
Conditional Statements
A type of coding instruction that tells the computer to compare values and expressions, and to make decisions. A conditional statement tells a program to perform an action based on whether the condition is true or false, often using the command IF, THEN, and ELSE.
Example
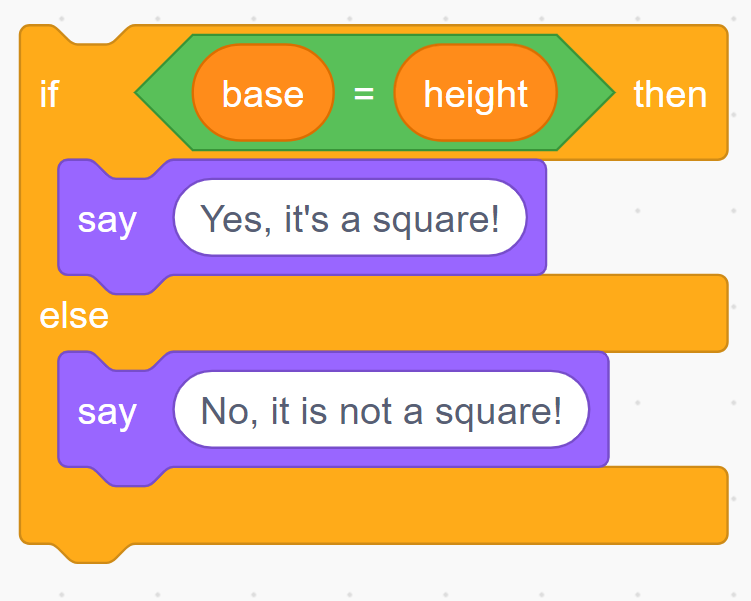