C3.2 Read and alter existing code, including code that involves conditional statements and other control structures, and describe how changes to the code affect the outcomes and the efficiency of the code.
Skill: Reading Code
Reading code involves interpreting blocks or commands to make predictions about its outcome. Reading code is also an important part of debugging to identify and correct errors of why the code is not working or getting the desired outcome.
When reading code, the student should notice, among other things, repetitions of commands or blocks that could be more efficiently expressed as a loop. For example, students can read the following code and identify the pattern:
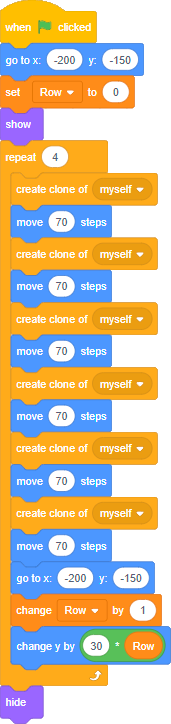
Note also that there are several ways to make code more efficient (using variables to represent values that are repeated in various places in the code, for example).
Skill: Altering Code
One of the reasons to alter code is to save time by not starting from scratch but to use existing code to build something new. Altering code can also be done to make the code more efficient and reduce runtime or to demonstrate that there is more than on way to use code to represent a situation. Another reason to alter code is to fix errors and make it functional, a process called debugging.
For example, the student could start with a code that makes a sprite move around the screen. The student's job would be to create interactive elements when the sprite comes into contact with other objects. Here is an example of a possible interaction:
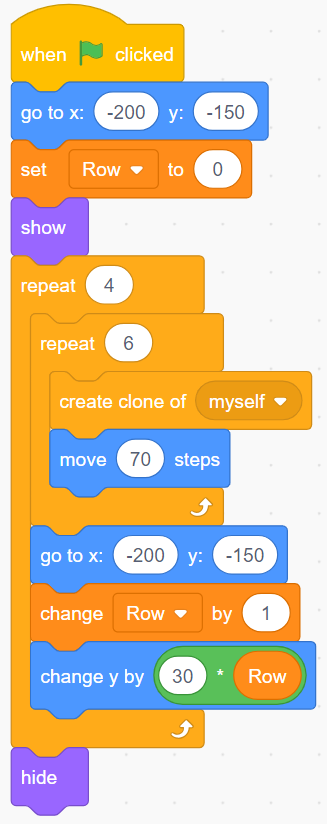
Skill: Describing the Impact of Altering Code
As much as trial and error is an important part of creating code, it is important that the student be able to justify their choices of changes to an existing code by making predictions about the effect of the changes on the outcome of the code. This is also important when altering code to make it efficient, as it is possible to introduce changes that have an unintended or negative impact on the final outcome.
For example, students could use their knowledge of integers to write the effect of a sign change (+/-) on displacements in the following code:
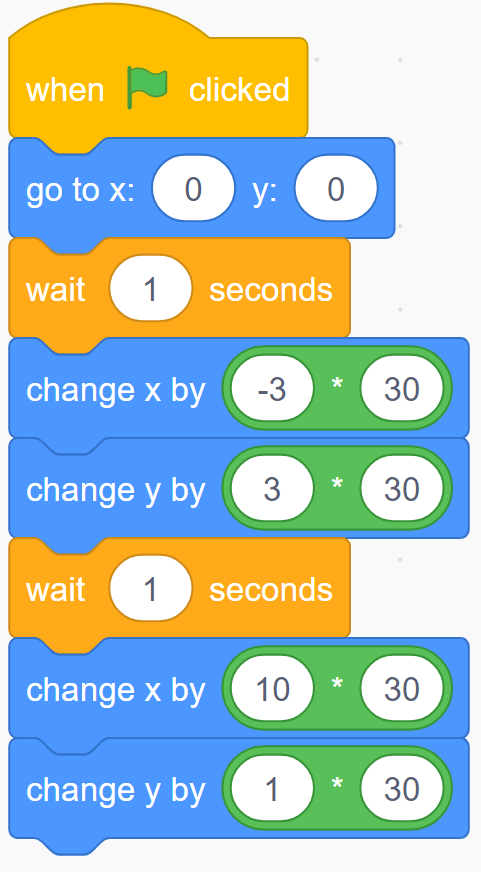