C3.1 Solve problems and create computational representations of mathematical situations by writing and executing efficient code, including code that involves events influenced by a defined count and/or subprogram and other control structures.
Skill: Computational Problem Solving
Coding can be used to automate tasks and to represent and solve problems. By its very nature, coding lends itself well to trial-and-error learning, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "What will happen if…?".
In addition, the question "what will happen if…?" allows one to start a conversation about conditions and conditional statements. For example, in a probability problem, one might ask "what will happen if I roll a die 1,000 times?" or even "how many times will I get 2 by rolling a die 1,000 times?" Given the time required to perform this experiment manually, code becomes an efficient way to solve such a problem.
Skill: Representing Mathematical Situations Computationally
Coding can be used as a mathematical learning tool to represent and manipulate complex mathematical situations.
For example, to calculate the mean of a set of data, the student will need to be familiar with how to perform this calculation in order to choose the right commands or blocks to achieve this goal. Here is an example of code that would calculate a mean:
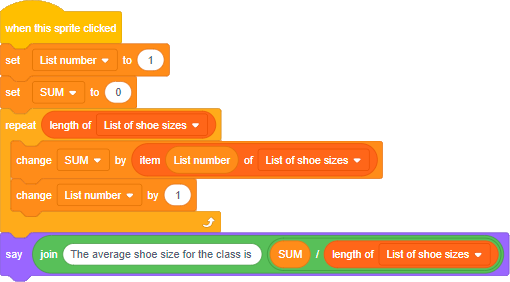
In this example, the calculation of the mean is represented, in the last line of code, as the sum of the data divided by the length of the list.
Skill: Writing Code
Writing and altering a code involves sequencing instructions in a specific order, following the syntax of a programming language. Writing code can be similar to writing text. Pseudocode is writing the instructions for a code in no particular program language.
For example, some code will use very complex sequences that, in the body of the main code, may represent cluttered and difficult-to-read code. Using a subprogram allows you to maintain some structure free of the main code by calling on the complex sequences when the need arises.
Here is an example of a subprogram that has been transformed into a custom block.
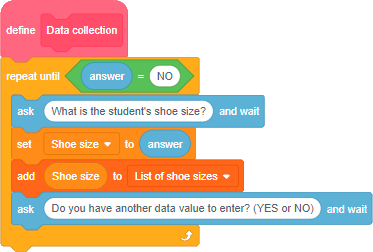
Once this block is created, the main code would look like this:
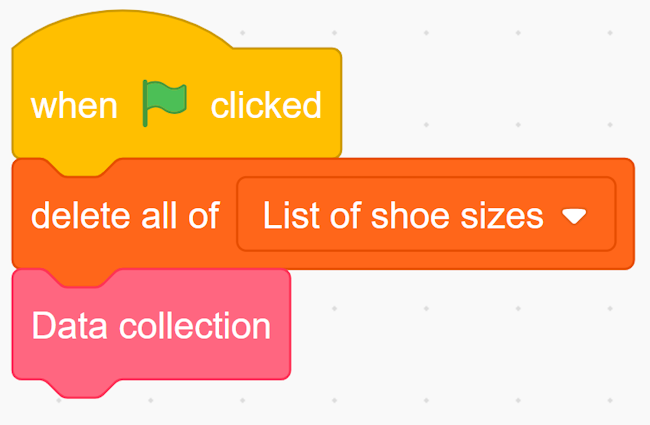
There are many ways to call a subprogram, and the program or language in which the code is written will affect this process.
Skill: Executing Code
Execution of code is when the code is read and compiled by the coding program and is referred to as the output. In block coding, the execution of a code is often done by means of a button in the interface, while text-based programming languages require precise compilation program that essentially translates the code from the programming language to the machine language (for example, binary code) so that it can execute the code. This also applies to robotic devices.
Some examples of run buttons for block-based coding program

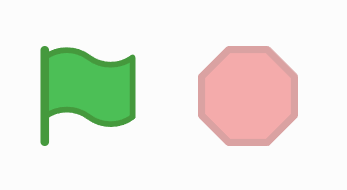
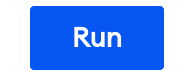
Knowledge: Subprograms
A subprogram is a small set of instructions that performs a simple task outside the main code. Subprograms can be combined in a main program to accomplish a large task using simple steps.
For example, it is possible to create a custom block that, when executed, calls a series of specific instructions (a subprogram). The first step is to create a block with two adjustable parameters (for example, base and height). In this case, the "rectangle" block sends the message to start executing the code below the "define: rectangle" block. This ensures that a rectangle of any size can be generated anywhere on the canvas, because the rectangle has been defined. Once the rectangle has been drawn, the main code continues.
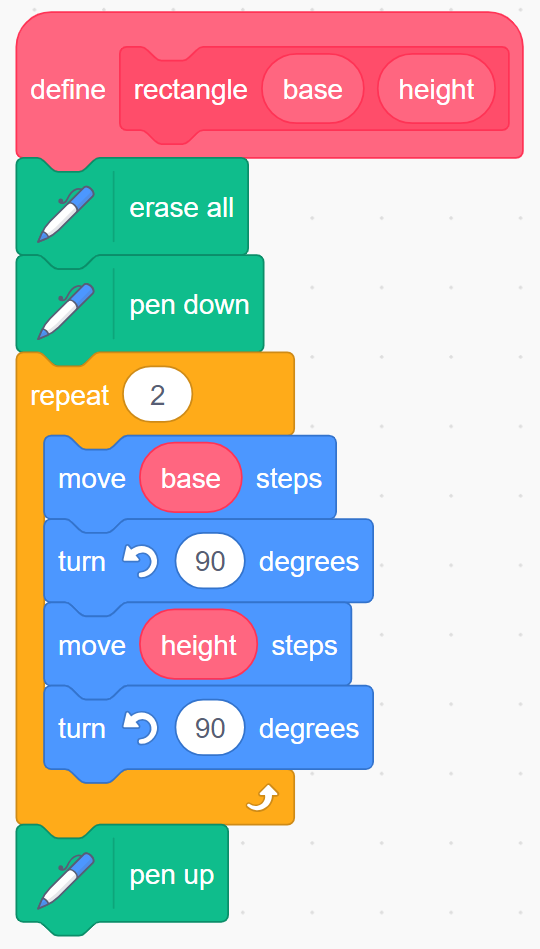
To insert the rectangle, simply add the new "rectangle" block to the code, and specify the base and height values.
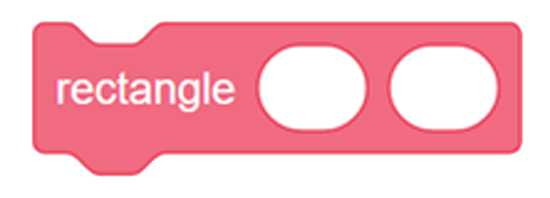
Note: The way to access subprograms depends on the programming language chosen.
Knowledge: Events and Structures (Prior Knowledge)
Sequential Events
A set of instructions executed one after the other.
Example

Simultaneous Events
Several events that occur at the same time, also called “concurrent” events.
Example
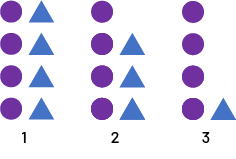
Recurring Events
Repeating events. In coding activities, loops are used in code to repeat instructions.
Example
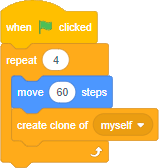
Nested Events
Control structures placed inside other control structures. For example, loops occurring inside loops or a conditional statement being evaluated inside a loop.
Example
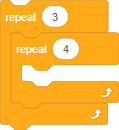
Conditional Statements
A conditional statement is a type of coding command that instructs the program to compare values and expressions and then make decisions based on whether the condition is true or false.
Conditional statements are first present when the execution of an event or a sequence of code depends on the achievement of a specific condition. Boolean values (true or false) are often used and associated with terms such as if, else, until and when.
Example
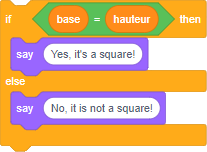
Code Efficiency
Writing functional code using a minimum of blocks or commands.
Example
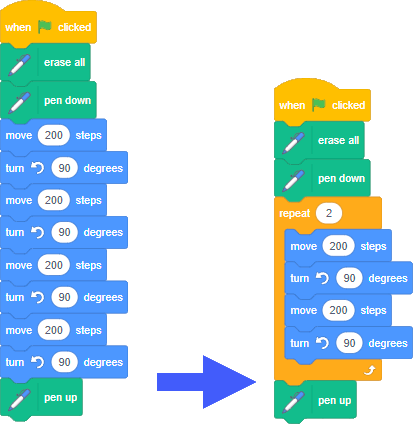