C3.1 Solve problems and create computational representations of mathematical situations by writing and executing code, including code that involves the analysis of data in order to inform and communicate decisions.
Skill: Solving Problems in a Computational Way
Coding can be used to automate tasks and visualize mathematics to facilitate problem solving. By its very nature, coding lends itself well to trial and error, giving students the opportunity to solve problems by learning from their mistakes. Therefore, the student can use the questioning "what will happen if…?".
For example, data collection and analysis can be demanding if done manually, but with the help of code, it is possible to collect data quickly and compile it into a list or spreadsheet, which also makes it easier to analyze.
Skill: Representing Mathematical Situations in Computational Ways
Coding can be used as a representational tool in the same way as manipulatives. By using written blocks or commands, very complex mathematical situations can be modelled and manipulated visually, which can make very abstract concepts concrete.
For example, in a spreadsheet, it is possible to represent a multi-step calculation with a single logical command. In this example, a multi-term addition is represented by the SUM command, followed by the cells to be added.
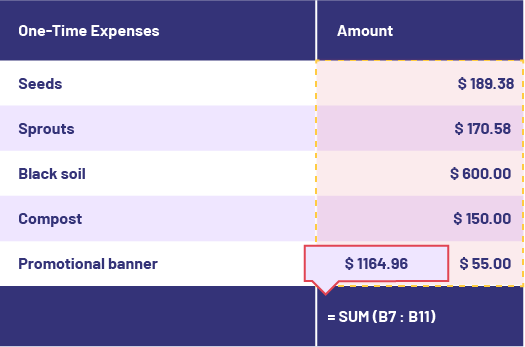
Skill: Writing Efficient Code
Writing or editing efficient code involves placing instructions in a specific order, following the syntax of a programming language and using a minimum number of blocks or commands to achieve the desired outcome. Writing code can be similar to writing text. Pseudocode writing, on the other hand, involves writing code directives in the familiar language. Block-based coding can make it easier to follow syntax by using different block shapes and colours.
For example, conditional cell formatting in a spreadsheet requires a few lines of code, but can make data analysis or outlier identification very easy and efficient. In this example, we see two conditions for the color of cells D12 through D15 in a spreadsheet.
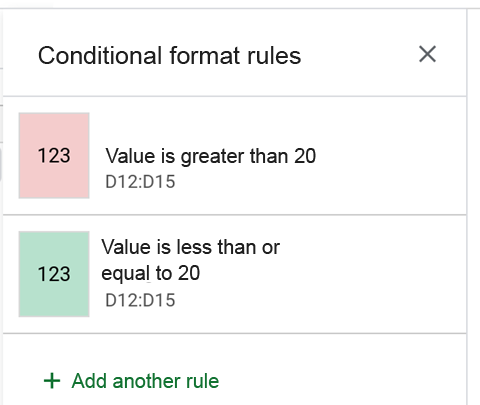
Note: To capture the conditional formatting, right click on the cell and choose "conditional formatting"
We could also represent this situation with pseudocode, that is :
For D12:D15
IF (value) < 20 OR (value) = 20
THEN color = GREEN
IF NOT color = RED
Skill: Executing Code
Code execution is the step where the code sequence is read and compiled by the computer. It is at this stage that a functional code will give the desired outcome (and a non-functional code will give a different outcome or no outcome). In block-based coding, code execution is often done by means of a button in the interface, whereas text-based programming languages require precise compilation software that essentially translates the code from the programming language to the machine language (for example, binary code) so that the machine can execute the code. This also applies to robotic devices.
Some examples of run buttons for block-based coding software:
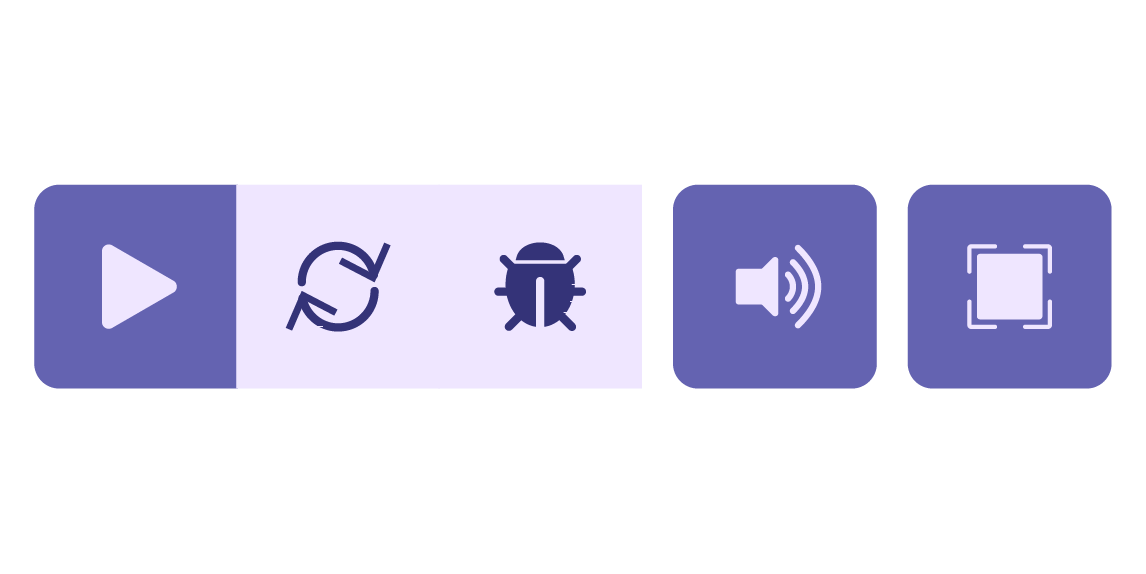
Run buttons in MakeCode.
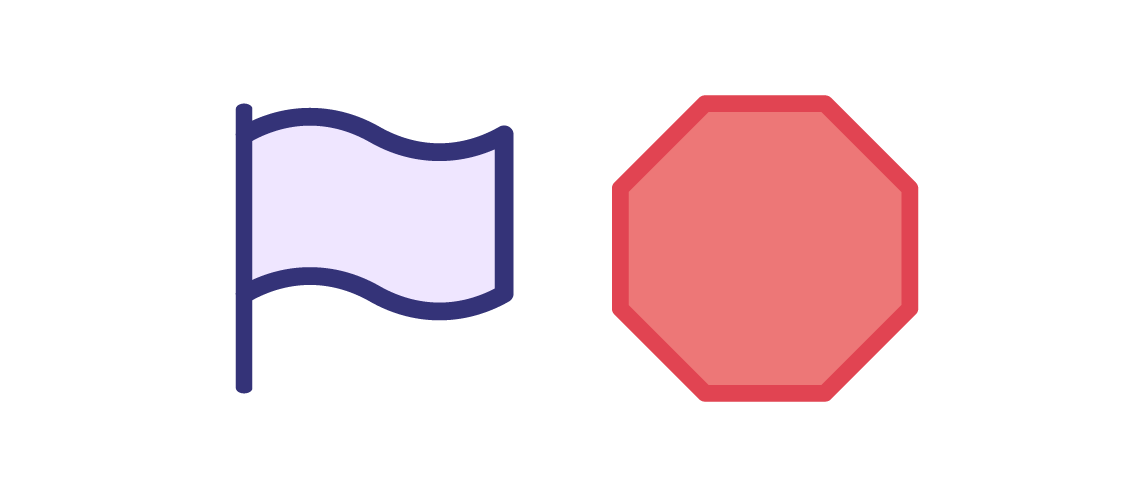
Run buttons in Scratch.
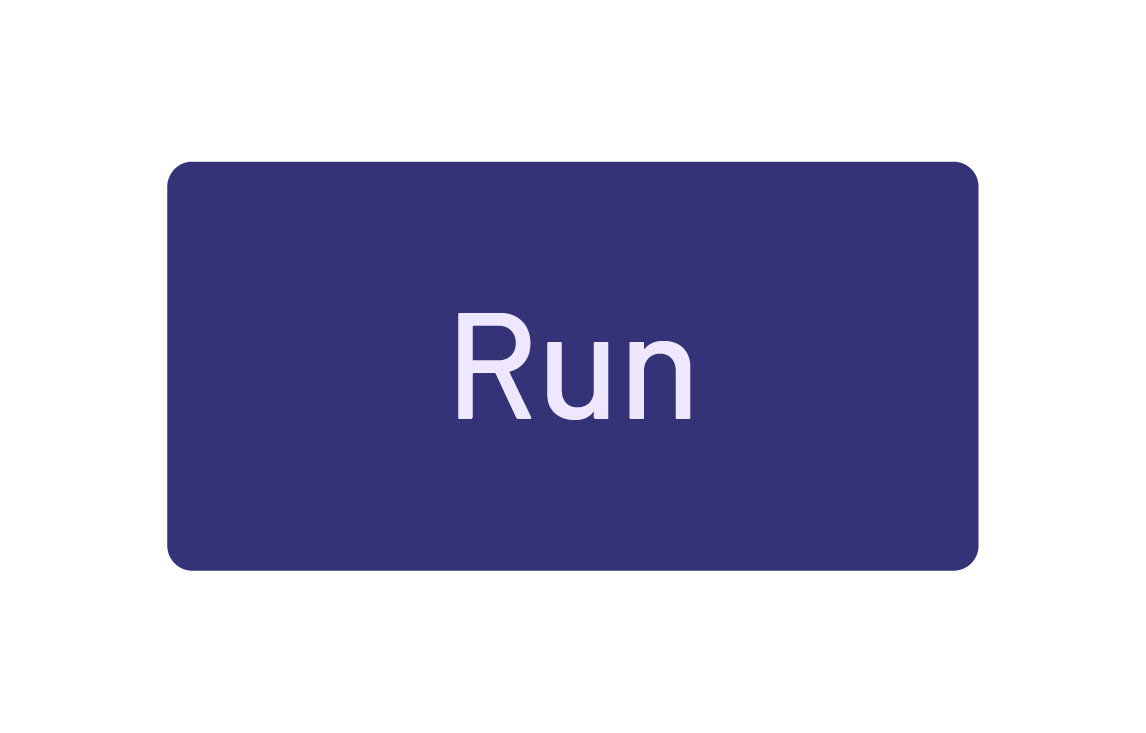
Python compilation software "Programiz" run button.
Skill: Analyzing Data with a Code
In order to make good decisions, you need good data. Coding software and microcontrollers (Arduino, Micro:bit, etc.) automate the collection and analysis of data, which enables decision-making and provides good arguments to support these decisions. Data entry can be done in several ways; for example, code could be written to enable data collection through a form that compiles the data into a spreadsheet. Most spreadsheets accept codes to facilitate data analysis.
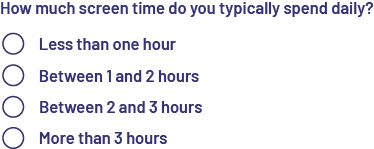
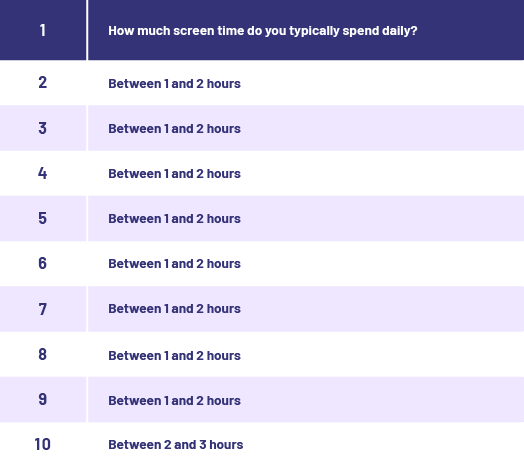
Raw data can be turned into a relative-frequency table, and conditional formatting can be applied to cells to highlight specific data or trends.
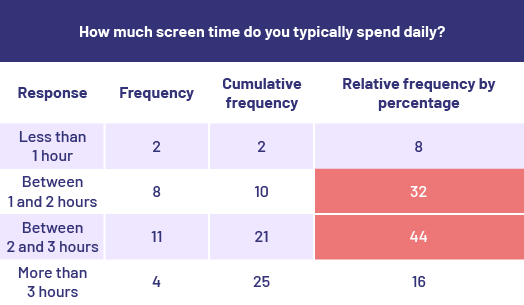
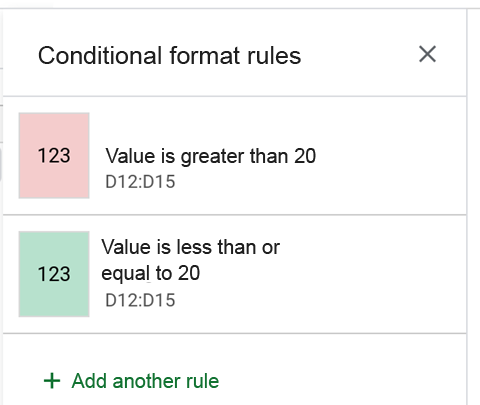
Note: To capture the conditional formatting, right click on the cell and choose "conditional formatting"
Sensors take it one step further. Many microcontrollers have sensors installed or plugged in that allow for data collection. These microcontrollers can be found everywhere in a student's daily life - the smart thermostat that acts as a switch that activates the ventilation system when a temperature is reached, or the accelerometer on a smartphone or smartwatch that determines the number of steps a person takes in a day to determine the distance traveled. Discussions about the ways in which data is collected and analyzed through coding will allow the student to further explore the limits of what is possible to accomplish with code.
Knowledge: Events and Structures (Prior Knowledge)
Sequential Events
A set of instructions executed one after the other, usually from top to bottom or from left to right on a screen.
Example
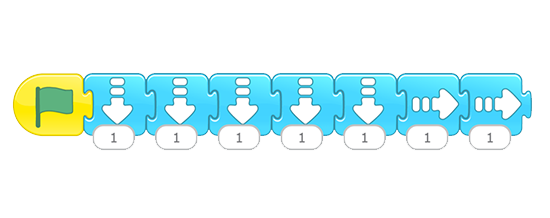
Concurrent Events
Several events that occur at the same time.
Example
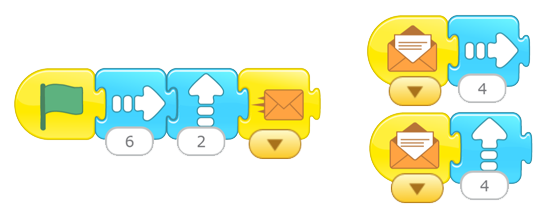
Recurring Events
Something that happens over and over again. In coding, loops are used to repeat instructions.
Example
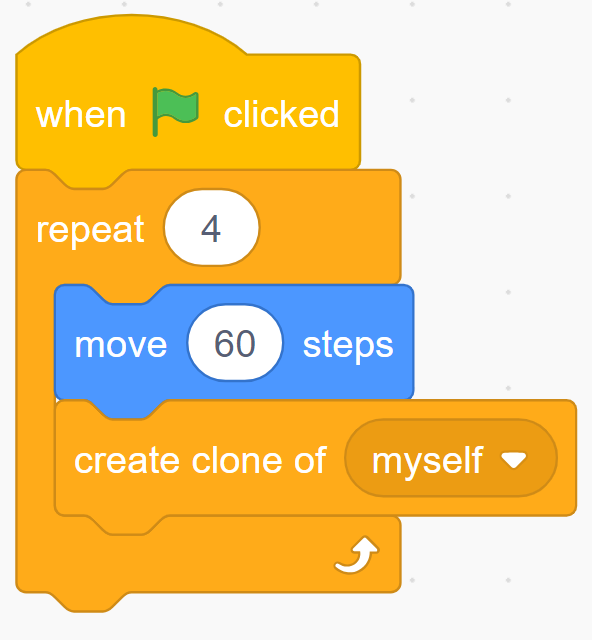
Nested Events
Control structures placed inside other control structures. For example, loops occurring inside loops or a conditional statement being inserted inside a loop.
Example
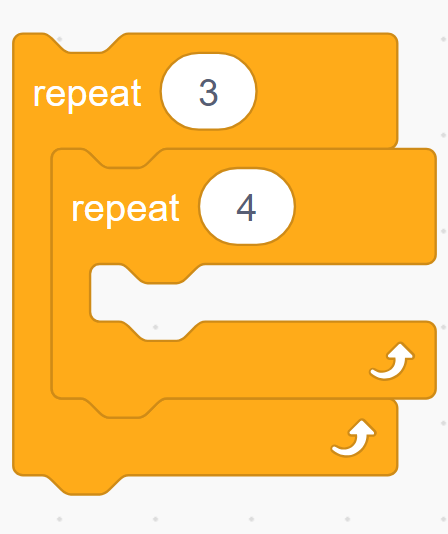
Conditional Statements
A type of coding instruction that tells the computer to compare values and expressions and make decisions. A conditional statement tells a program to perform an action based on whether the condition is true or false, often using the vocabulary IF, THEN, and ELSE.
Example
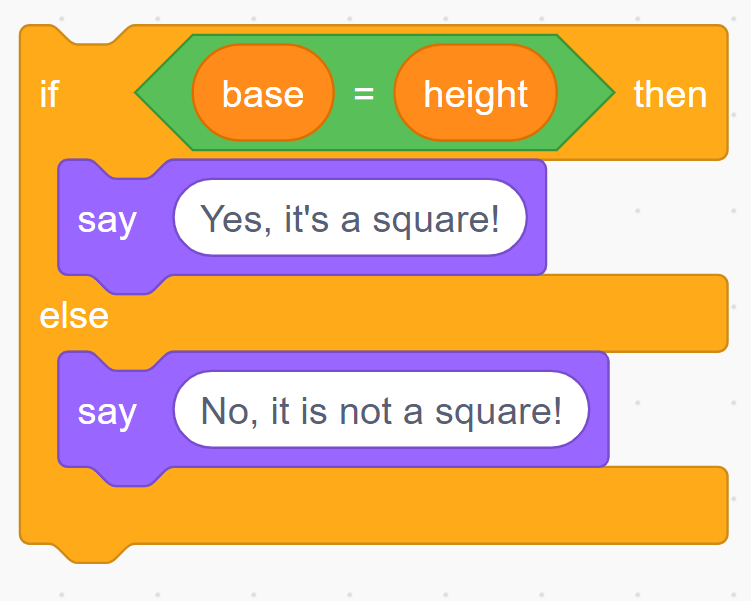
Code Efficiency
The practice of writing functional code using a minimum of blocks or commands.
Example
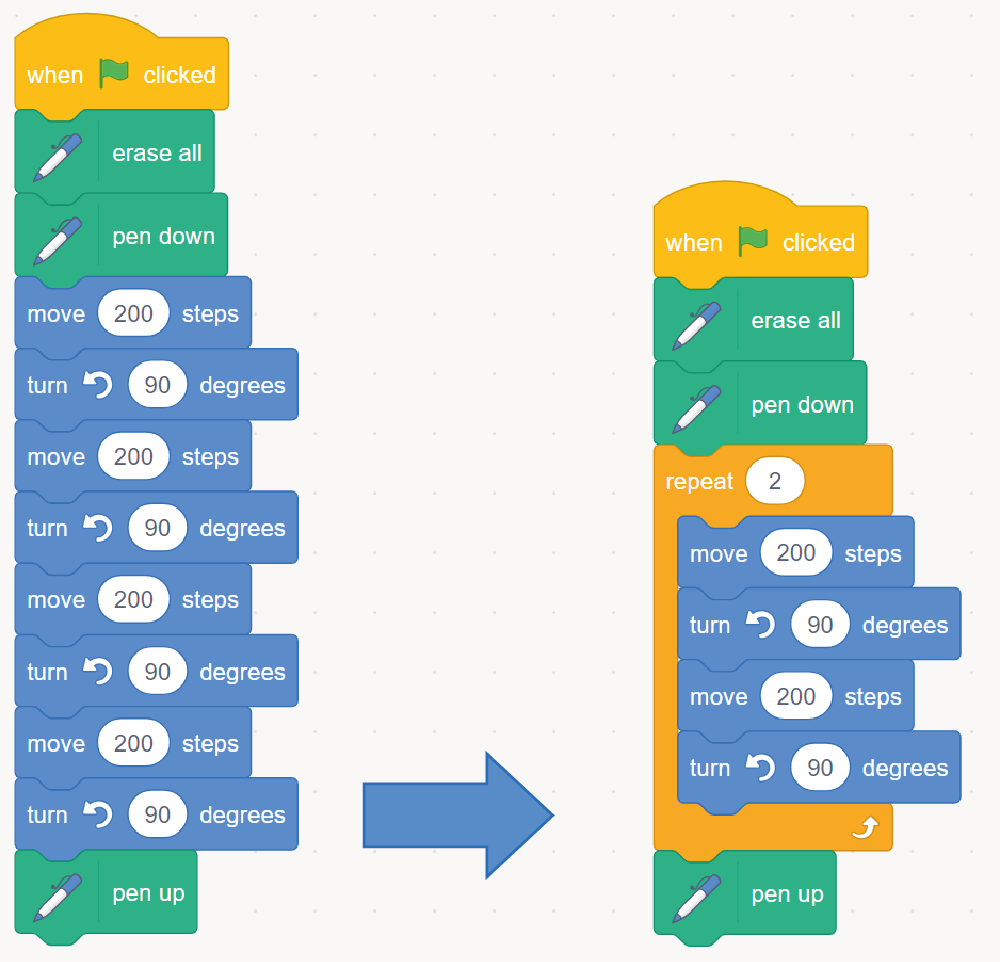
Subprograms
A small set of instructions that performs a simple task. Subprograms can be combined in a main program to accomplish a large task using simple steps.
Example
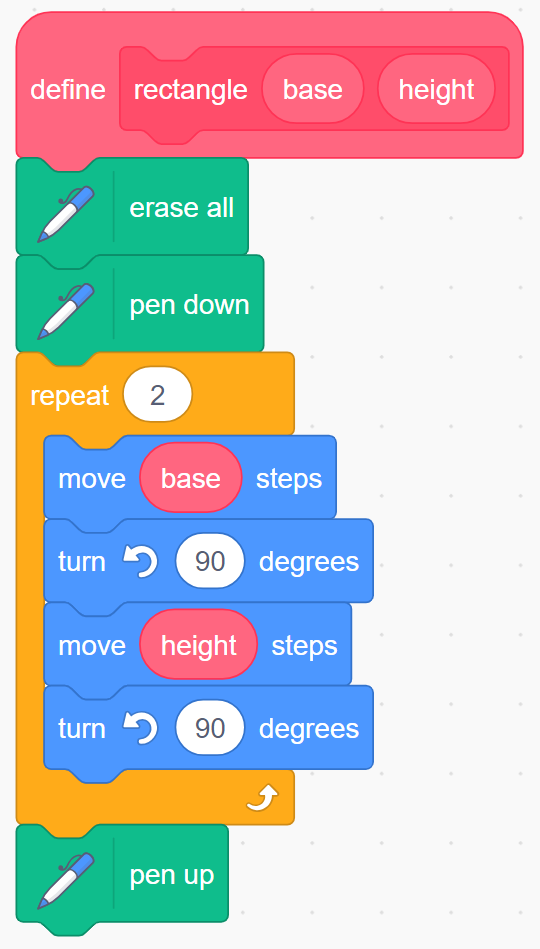
To insert the rectangle, simply add the new "rectangle" block to the code, and specify the base and height values.
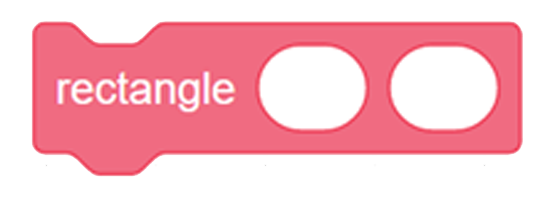
Defined Count
Number of times instructions are repeated based on a predefined value or until a condition is met.
Example
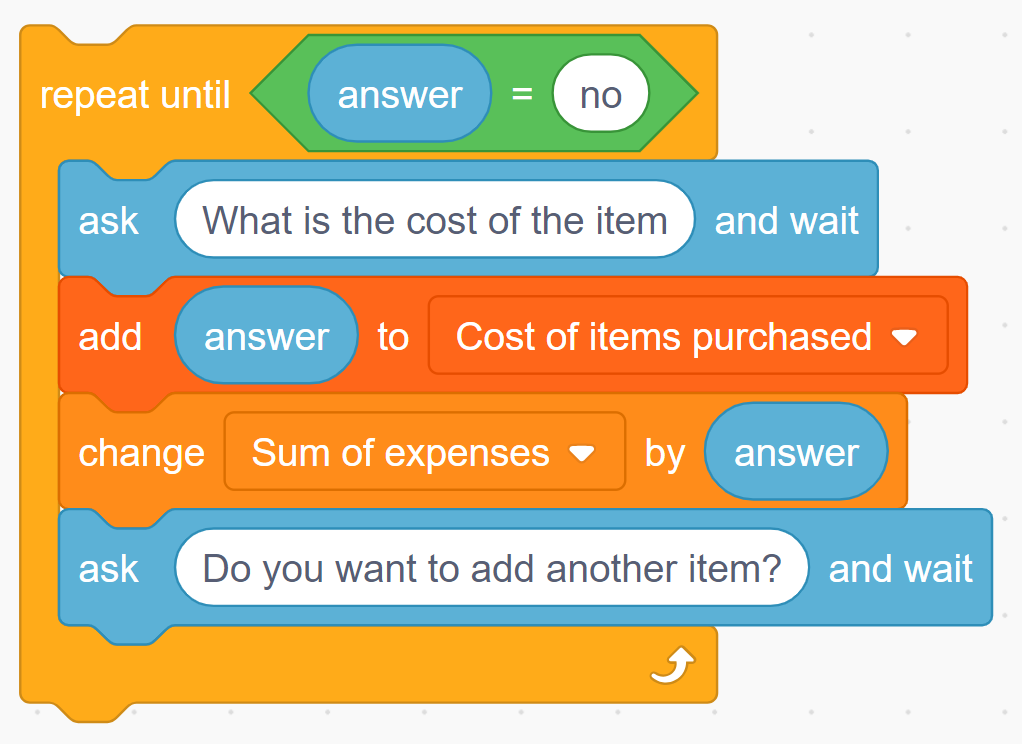